Sanitize data and prevent SQL injections in php
Posted by Warith Al Maawali on Jun 7, 2013 in Blog, Source-Codes | 4 comments
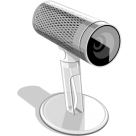
The reason for adding this blog is sometimes when I code in php I normally forget that data has to be sanitized before executing to prevent or XSS attacks. The simplest way to make SQL injection hard is to use either Mysqli or PDO prepare statements – Examples – as it keeps the SQL and data inputs completely separated. However if you need to use them on normal php statements here are good methods that I use.
Methods:
Integer values:
// To SANITIZE Integer value use $var=(filter_var($var, FILTER_SANITIZE_NUMBER_INT)); //example: $theNumber="983928/2ddo@3233'0 or 1 '%^33)_23@''''$9123!@~#"; $theNumber=(filter_var($theNumber, FILTER_SANITIZE_NUMBER_INT)); echo $theNumber; //cleaned out put will be: 983928232330133239123
Email values:
//To SANITIZE email query value use $var=(filter_var($var, FILTER_SANITIZE_EMAIL)); //example: $theEmail="warith@d\igi7/7.com"; $theEmail=(filter_var($theEmail, FILTER_SANITIZE_EMAIL)); echo $theEmail; //cleaned out put will be: warith@digi77.com;
String values:
//To SANITIZE String value use function StringInputCleaner($data) { //remove space bfore and after $data = trim($data); //remove slashes $data = stripslashes($data); $data=(filter_var($data, FILTER_SANITIZE_STRING)); return $data; } //example: $myString="Welcome <script> alert('\Hi digi77.com')</script> here"; ; $myString=StringInputCleaner($myString); echo $myString; //cleaned out put will be: Welcome alert('Hi digi77.com') here
Sql statments:
//To SANITIZE Sql query value use function mysqlCleaner($data) { $data= mysql_real_escape_string($data); $data= stripslashes($data); return $data; //or in one line code //return(stripslashes(mysql_real_escape_string($data))); } //example: $insert="delete from vbtube_tubes WHERE tubeid =$row5[0]"; $insert= mysqlCleaner($insert); mysql_query($insert);
Quik refrence:
- mysql_real_escape_string //—> used when inserting into database.
- htmlentities() //—> used when outputing data into web page.
- htmlspecialchars() //—> used if u want to display the html tags and not execute them.
- strip_tags() //—> used when remove html tags.
- addslashes() //—> used when u need to add extra front slash for every back slash.
- stripslashes() //—> remove slashes.
More Santizing functions:
- FILTER_SANITIZE_NUMBER_FLOAT
- FILTER_SANITIZE_SPECIAL_CHARS
- FILTER_SANITIZE_STRING
- FILTER_SANITIZE_URL
- FILTER_SANITIZE_ENCODED

The following two tabs change content below.

Crypto currency , security , coding addicted I am not an expert or a professional I just love & enjoy what I am addicted onðŸ¤

Latest posts by Warith Al Maawali (see all)
- Apple iOS Mail Client leaking highly sensitive information - December 27, 2019
- Validating VPN nodes - November 3, 2019
- Migrating from php 5.6 to 7.3 - November 1, 2019
- Linux Kodachi 8.27 The Secure OS - October 20, 2013
- Migrating from Vbulletin to Burning board - March 27, 2016
SQL injection is a code injection technique, used to attack data-driven applications, in which malicious SQL statements are inserted into an entry field for execution (e.g. to dump the database contents to the attacker).
I caution everyone against using
mysql_real_escape_string()
. You really should be using Prepared Statements instead.Also, your last example (“Sql statements”) appears to be very misguided. There isn’t some magic function that predicts what you want and saves you from hackers. You have to be very explicit. The best way to do this is to separate your data from the instructions that operate on said data.
This advice is pretty dangerous. I’d strongly recommend revising it. Our team publishes a lot of blog posts about security engineering if you’re interested in reading up on the subject.
Dear Paragon Initiative Enterprises,
If you have read the blog from the beginning you would have seen that I have recommended to use Prepared Statements to start with.
Thank you for your advice please do share good example if you have.
Dear Walid,
As far as I know magic quotes will help but not prevent so the answer is Yes it can be injected because magic quotes uses PHP’s addslashes() function which is not unicode aware this means multi-byte characters are not escaped.
I’m wondring if the website can be injected when the Magic Quotes in server is ON